The figure below shows the Zing block diagram. Out of this diagram, the data storage facility is built around four concepts: type definitions, data sources, generated code and rights management.
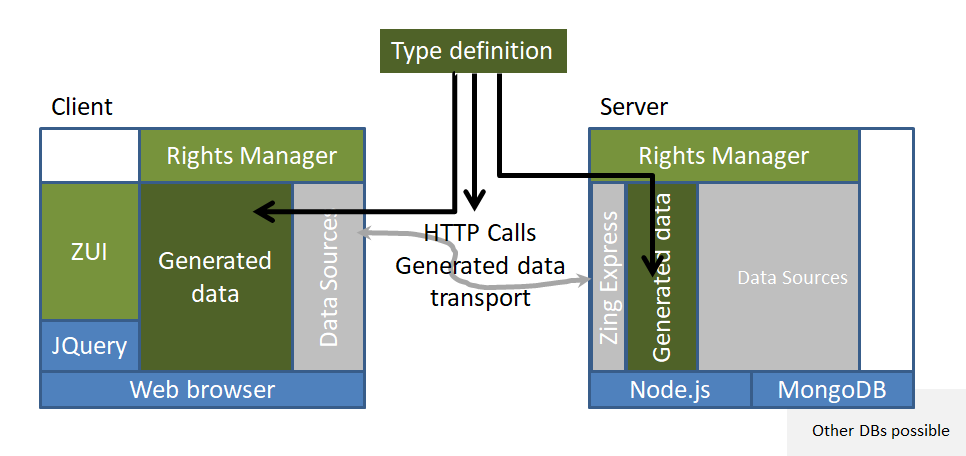
The purpose of the data storage system is to maintain consistent data models between the client and the server. This system ensures the following:
- Data definitions are consistent between client and server and they are consistent with the TypeScript class system.
- The communication of data between the client and the server is more or less transparent to the programmer.
- The communication between code and HTTP and between code and MongoDB is all handled automatically.
- Caching data objects in the client that are actually stored on the server is handled automatically and accurately.
Type definition
The Zing type definition system takes type definitions specified in JSON and translates them into generated TypeScript code. This generated code is used to bind the specific types required for a particular application to the more general communications and storage tools.
- See Type Generation
Data Sources
The TypeScript class DataSource is the basis for all data management in Zing. DataSource is an abstract class that defines the methods for all data sources. An application both on the client and the server side performs its operations through DataSources. The API for DataSources is uniform but the functionality is not.
HTTPDataSource
A web client primarily uses the HTTPDataSource. This class translates all data operations into HTTPS requests on the server. The most common way for a client to set up an HTTPDataSource is as follow:
let httpSource = new HTTPDataSource(window.location.origin+"/");
A client web application generally starts by loading a page that contains JavaScript to start the application. This is generally loaded from the server where the application resides. The “window.location.origin” contains the URL of the website where the current page came from. The code shown above will create an HTTPDataSource that will send all of its HTTP requests to that location. Other parameters are possible for other configurations of server and web pages.
CacheDataSource
Just using the HTTPDataSource as is can be very inefficient. Each time an object or a search is requested a round trip to the server is performed through an HTTP request. The CacheDataSource alleviates that problem. The CacheDataSource retains an in-memory cache of previously retrieved object and/or search results. If the desired information is already in memory it is returned without requiring an HTTP request. If changes have been made to objects their cached value is discarded so that future request will return to the server for a fresh copy. Warning: this cache is not aware of other clients simultaneously accessing those same objects from the same server. It cannot prevent cache problems between clients. A CacheDataSource is set up as follows.
let source = new CacheDataSource( another data source ); or let httpSource = new HTTPDataSource(window.location.origin+"/"); let source = new CacheDataSource(httpSource); DataObj.globalSource = source;
CacheDataSource takes another data source as its parameter. It passes all of its requests through to the parameter source and caches the results. The most common usage is shown in the bottom three lines. An HTTPDataSource is created that references the server and that is then passed to the CacheDataSource. The last line shows the source being assigned to the DataObj.globalSource. This tells the DataObj class where to go to have data storage services performed.
MongoDataSource
This data source is used by servers to access a MongoDB database where data objects are stored. The DataSource methods that MongoDataSource inherits provide the actual data functionality. A MongoDataSource is created as follows:
let source = new MongoDataSource( "userName:password@host", port,database )
The first parameter specifies how to access the MongoDB server. You will need a userName and password for that server as well as the domain name or IP address where that server is located. The port is the IP port number on which the server is listening. A MongoDB server can hold multiple databases. The database parameter is the name of the database to be used by your server.
DataObj
As described in the section on Type Generation, the DataObj class is the superclass of all data objects known to the Zing type system. For the most part all data manipulation is performed through the DataObj class or its subclasses defined by the Type Generation system.
- See DataObj methods
Only a few of the DataObj methods are accessed directly. Most of the operations are methods of the generated types. These have appropriate type declarations generated to ensure correctness.
DataSource
A programmer will rarely access the DataSource methods directly. These are usually called by the methods defined on the various generated types.
Rights management
Zing has a rights management mechanism that is integrated into the DataSource methods. This mechanism is called both on the client side and the server side to verify that the currently logged in use has the right to perform the specified operation on the data. One can create separate rights management for the client and the server where needed. In most cases you want the same rights imposed on both size.
Checking rights on the client side allows for early inexpensive verification of rights before contacting the server. Checking rights on the server is most important because that protects your data against malicious code built outside of the Zing system.